Running the quickstart via the API#
Flow360 can be automatically driven via our powerful Python API. All the case setup parameters available in the WebUI are available in the Python API.
By starting from the same evtol_quickstart_grouped.csm file as we have downloaded in the above geometry upload section, The EVTOL quickstart case shown previously can be easily launched using the following code:
1# Import necessary modules from the Flow360 library
2from matplotlib.pyplot import show
3
4import flow360 as fl
5from flow360.examples import Airplane
6
7# Step 1: Create a new project from a predefined geometry file in the Airplane example
8# This initializes a project with the specified geometry and assigns it a name.
9project = fl.Project.from_geometry(
10 Airplane.geometry,
11 name="Python Project (Geometry, from file)",
12)
13geo = project.geometry # Access the geometry of the project
14
15# Step 2: Display available groupings in the geometry (helpful for identifying group names)
16geo.show_available_groupings(verbose_mode=True)
17
18# Step 3: Group faces by a specific tag for easier reference in defining `Surface` objects
19geo.group_faces_by_tag("groupName")
20
21# Step 4: Define simulation parameters within a specific unit system
22with fl.SI_unit_system:
23 # Define an automated far-field boundary condition for the simulation
24 far_field_zone = fl.AutomatedFarfield()
25
26 # Set up the main simulation parameters
27 params = fl.SimulationParams(
28 # Meshing parameters, including boundary layer and maximum edge length
29 meshing=fl.MeshingParams(
30 defaults=fl.MeshingDefaults(
31 boundary_layer_first_layer_thickness=0.001, # Boundary layer thickness
32 surface_max_edge_length=1, # Maximum edge length on surfaces
33 ),
34 volume_zones=[far_field_zone], # Apply the automated far-field boundary condition
35 ),
36 # Reference geometry parameters for the simulation (e.g., center of pressure)
37 reference_geometry=fl.ReferenceGeometry(),
38 # Operating conditions: setting speed and angle of attack for the simulation
39 operating_condition=fl.AerospaceCondition(
40 velocity_magnitude=100, # Velocity of 100 m/s
41 alpha=5 * fl.u.deg, # Angle of attack of 5 degrees
42 ),
43 # Time-stepping configuration: specifying steady-state with a maximum step limit
44 time_stepping=fl.Steady(max_steps=1000),
45 # Define models for the simulation, such as walls and freestream conditions
46 models=[
47 fl.Wall(
48 surfaces=[geo["*"]], # Apply wall boundary conditions to all surfaces in geometry
49 ),
50 fl.Freestream(
51 surfaces=[
52 far_field_zone.farfield
53 ], # Apply freestream boundary to the far-field zone
54 ),
55 ],
56 # Define output parameters for the simulation
57 outputs=[
58 fl.SurfaceOutput(
59 surfaces=geo["*"], # Select all surfaces for output
60 output_fields=["Cp", "Cf", "yPlus", "CfVec"], # Output fields for post-processing
61 )
62 ],
63 )
64
65# Step 5: Run the simulation case with the specified parameters
66project.run_case(params=params, name="Case of Simple Airplane from Python")
67
68# Step 6: wait for results and plot CL, CD when available
69case = project.case
70case.wait()
71
72total_forces = case.results.total_forces.as_dataframe()
73total_forces.plot("pseudo_step", ["CL", "CD"], ylim=[-5, 15])
74show()
When launching you will see the following output:
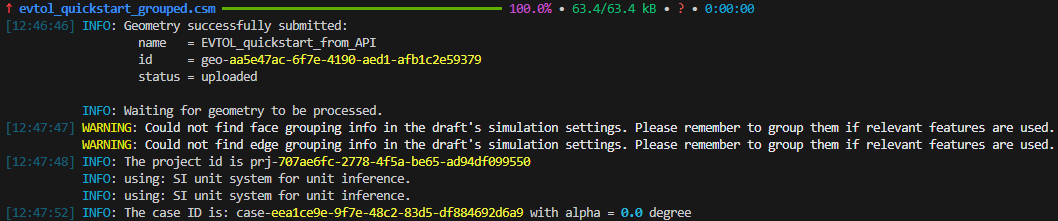
Output from running the API. Note that the IDs will be different when you run it.#
The script will wait for simulation to complete. After completition, it will plot CL and CD convergence plot:
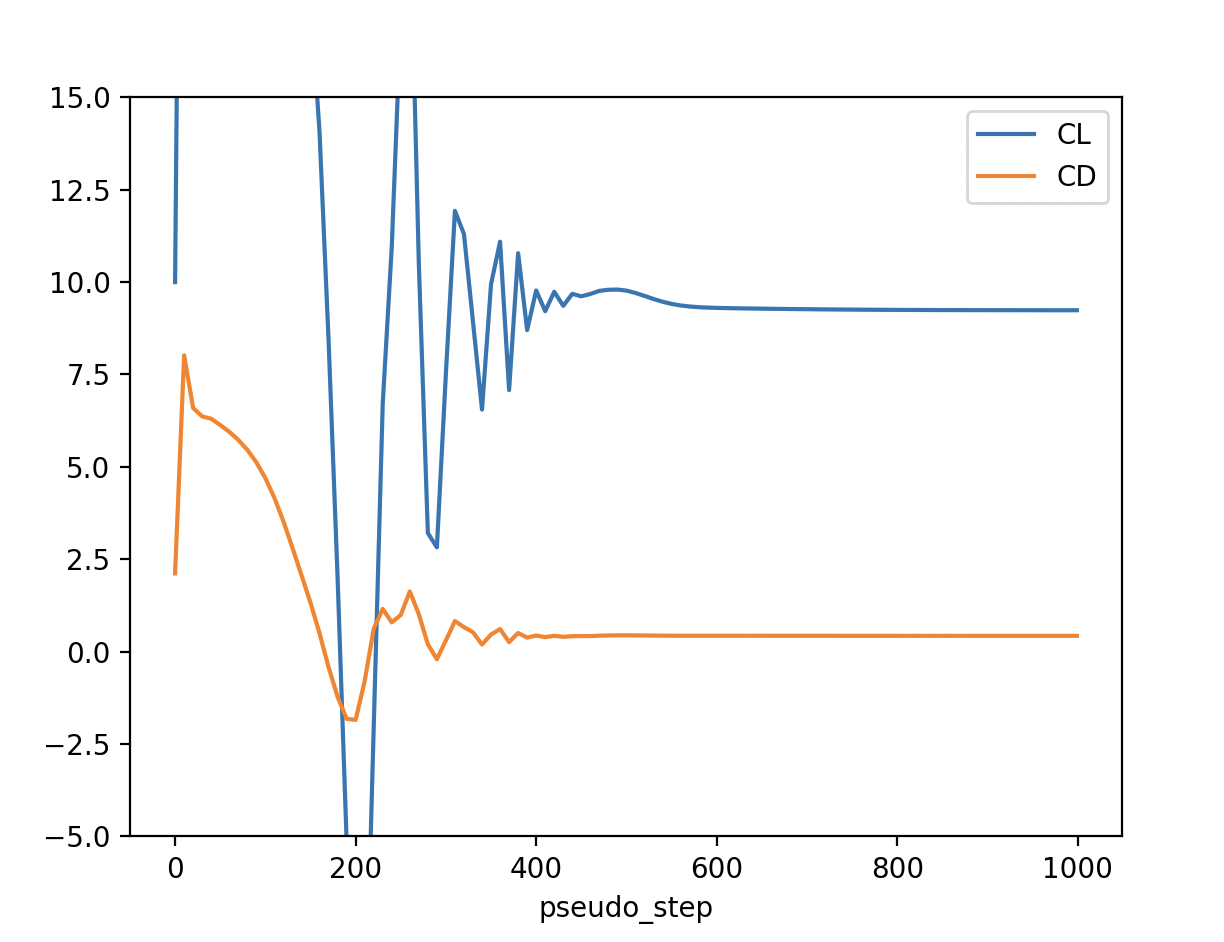
Force coefficient convergence plot.#
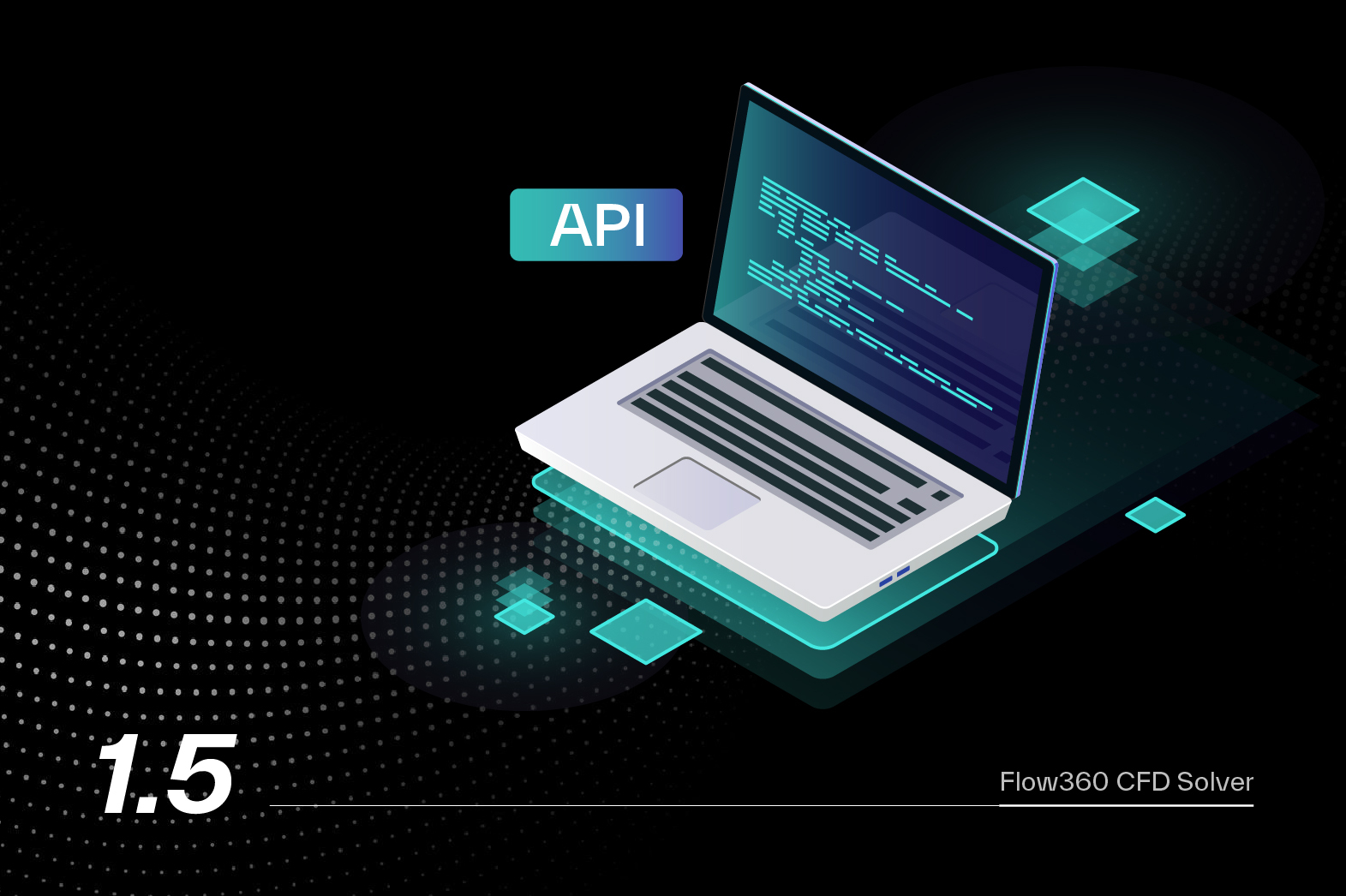