4. Python API Guide π§#
4.5. Installation#
Flow360 Python API (or also known as Python client) allows user to configure/submit/run/manage simulations using Python scripts. The latest release version of Flow360 Python API can be installed using pip:
pip install flow360
To install a specific version of Python API, for example 24.11.12:
pip install flow360==24.11.12
To install a specific Github branch of Python API, for example develop branch:
pip3 install git+https://github.com/flexcompute/Flow360.git@develop
Note that Flow360 Python API only supports Python versions between 3.9 and 3.12.
4.6. Configuring API key#
Most of the functionalities (like uploading mesh and submitting case) of Flow360 Python API requires setting up proper API key for authentication. The API key can be obtained from the Flow360 WebUI by clicking on your avatar on the top right of your workspace then clicking on your Account then Python Authentication. Please contact customer support for more information.
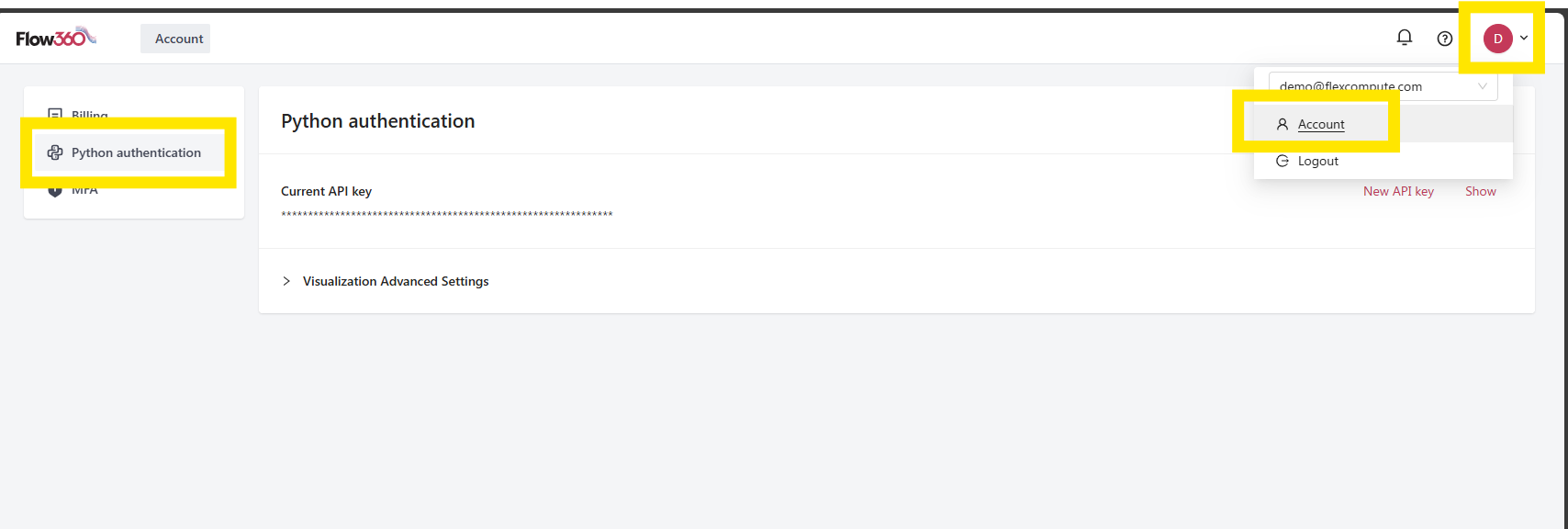
Fig. 4.6.1 Accessing your API key.#
To setup the API key authentication, you can run the following command: ``
In the command line. Run the following command.
flow360 configure --apikey YOUR_API_KEY_HERE
If you want to configure the API key on Windows, you can run the following command in the command prompt.
flow360 configure --apikey YOUR_API_KEY_HERE
In case the above command fails. A workaround is to set the API key with the following python script.
import flow360 as fl
fl.configure(apikey='YOUR_API_KEY_HERE')
4.7. Getting started π#
A quick example to use Flow360 Python API to upload a geometry file, specifying the parameters and run meshing and case is shown below:
1import flow360 as fl
2from flow360 import SI_unit_system, u
3from flow360.examples import Airplane
4
5# Step 1: Create a project from a geometry file
6project = fl.Project.from_file(Airplane.geometry,
7 name="Python Project from Geometry")
8geo = project.geometry # Access the geometry of the project
9
10# Step 2: Display available groupings in the geometry
11# (helpful for identifying group names)
12geo.show_available_groupings(verbose_mode=True)
13
14# Step 3: Group faces by a specific tag for easier reference
15# in defining `Surface` objects
16geo.group_faces_by_tag("groupName")
17
18# Step 4: Construct the simulation parameters under SI unit system
19with SI_unit_system:
20 # Define an automated far-field boundary condition for the simulation
21 far_field_zone = fl.AutomatedFarfield()
22
23 # Set up the main simulation parameters
24 params = fl.SimulationParams(
25 meshing=fl.MeshingParams(
26 defaults=fl.MeshingDefaults(
27 boundary_layer_first_layer_thickness=0.001,
28 surface_max_edge_length=1
29 ),
30 volume_zones=[far_field_zone],
31 ),
32 reference_geometry=fl.ReferenceGeometry(),
33 operating_condition=fl.AerospaceCondition(
34 velocity_magnitude=100,
35 alpha=5 * u.deg,
36 ),
37 time_stepping=fl.Steady(max_steps=1000),
38 models=[
39 fl.Wall(
40 surfaces=[geo["*"]], # Selecting all grouped faces
41 name="Wall",
42 ),
43 fl.Freestream(
44 surfaces=[far_field_zone.farfield],
45 name="Freestream",
46 ),
47 ],
48 outputs=[
49 fl.SurfaceOutput(
50 surfaces=geo["*"],
51 output_fields=["Cp", "yPlus"],
52 )
53 ],
54 )
55
56# Step 5: Run the simulation case with the specified parameters
57project.run_case(params=params, name="Case of Simple Airplane from Python")
Key concepts used in the above example:#
Project: The user interface to interact with cloud assets. Learn moreβ¦
Entities: The user can access entities (boundaries, edge etc) of cloud assets. Learn moreβ¦
Units: User can specify units for most of the parameters or ask the unit system to handle unit inference. Learn moreβ¦
4.8. Python API Example Library#
To explore usage of Flow360 Python API, you can refer to the example library where we demonstrate some of the common usage of Flow360 Python API.