Computing the scattering matrix of a device#
Note: the cost of running the entire notebook is larger than 1 FlexCredit.
This notebook will give a demo of the Tidy3D
ComponentModeler plugin used to compute scattering matrix elements.
For more integrated photonic examples such as the 8-Channel mode and polarization de-multiplexer, the broadband bi-level taper polarization rotator-splitter, and the broadband directional coupler, please visit our examples page.
If you are new to the finite-difference time-domain (FDTD) method, we highly recommend going through our FDTD101 tutorials. FDTD simulations can diverge due to various reasons. If you run into any simulation divergence issues, please follow the steps outlined in our troubleshooting guide to resolve it.
[1]:
# make sure notebook plots inline
%matplotlib inline
# standard python imports
import numpy as np
import matplotlib.pyplot as plt
import os
import gdstk
# tidy3D imports
import tidy3d as td
from tidy3d import web
Setup#
We will simulate a directional coupler, similar to the GDS and Parameter scan tutorials.
Letβs start by setting up some basic parameters.
[2]:
# wavelength / frequency
lambda0 = 1.550 # all length scales in microns
freq0 = td.constants.C_0 / lambda0
fwidth = freq0 / 10
# Spatial grid specification
grid_spec = td.GridSpec.auto(min_steps_per_wvl=14, wavelength=lambda0)
# Permittivity of waveguide and substrate
wg_n = 3.48
sub_n = 1.45
mat_wg = td.Medium(permittivity=wg_n**2)
mat_sub = td.Medium(permittivity=sub_n**2)
# Waveguide dimensions
# Waveguide height
wg_height = 0.22
# Waveguide width
wg_width = 0.5
# Waveguide separation in the beginning/end
wg_spacing_in = 8
# length of coupling region (um)
coup_length = 5.0
# spacing between waveguides in coupling region (um)
wg_spacing_coup = 0.07
# Total device length along propagation direction
device_length = 100
# Length of the bend region
bend_length = 16
# Straight waveguide sections on each side
straight_wg_length = 4
# space between waveguide and PML
pml_spacing = 1.2
Define waveguide bends and coupler#
Here is where we define our directional coupler shape programmatically in terms of the geometric parameters
[3]:
def tanh_interp(max_arg):
"""Interpolator for tanh with adjustable extension"""
scale = 1 / np.tanh(max_arg)
return lambda u: 0.5 * (1 + scale * np.tanh(max_arg * (u * 2 - 1)))
def make_coupler(
length,
wg_spacing_in,
wg_width,
wg_spacing_coup,
coup_length,
bend_length,
npts_bend=30,
):
"""Make an integrated coupler using the gdstk RobustPath object."""
# bend interpolator
interp = tanh_interp(3)
delta = wg_width + wg_spacing_coup - wg_spacing_in
offset = lambda u: wg_spacing_in + interp(u) * delta
coup = gdstk.RobustPath(
(-0.5 * length, 0),
(wg_width, wg_width),
wg_spacing_in,
simple_path=True,
layer=1,
datatype=[0, 1],
)
coup.segment((-0.5 * coup_length - bend_length, 0))
coup.segment(
(-0.5 * coup_length, 0),
offset=[lambda u: -0.5 * offset(u), lambda u: 0.5 * offset(u)],
)
coup.segment((0.5 * coup_length, 0))
coup.segment(
(0.5 * coup_length + bend_length, 0),
offset=[lambda u: -0.5 * offset(1 - u), lambda u: 0.5 * offset(1 - u)],
)
coup.segment((0.5 * length, 0))
return coup
Create Base Simulation#
The scattering matrix tool requires the βbaseβ Simulation (without the modal sources or monitors used to compute S-parameters), so we will construct that now.
We generate the structures and add a FieldMonitor so we can inspect the field patterns.
[4]:
# Geometry must be placed in GDS cells to import into Tidy3D
coup_cell = gdstk.Cell("Coupler")
substrate = gdstk.rectangle(
(-device_length / 2, -wg_spacing_in / 2 - 10),
(device_length / 2, wg_spacing_in / 2 + 10),
layer=0,
)
coup_cell.add(substrate)
# Add the coupler to a gdspy cell
gds_coup = make_coupler(
device_length, wg_spacing_in, wg_width, wg_spacing_coup, coup_length, bend_length
)
coup_cell.add(gds_coup)
# Substrate
(oxide_geo,) = td.PolySlab.from_gds(
gds_cell=coup_cell, gds_layer=0, gds_dtype=0, slab_bounds=(-10, 0), axis=2
)
oxide = td.Structure(geometry=oxide_geo, medium=mat_sub)
# Waveguides (import all datatypes if gds_dtype not specified)
coupler1_geo, coupler2_geo = td.PolySlab.from_gds(
gds_cell=coup_cell, gds_layer=1, slab_bounds=(0, wg_height), axis=2
)
coupler1 = td.Structure(geometry=coupler1_geo, medium=mat_wg)
coupler2 = td.Structure(geometry=coupler2_geo, medium=mat_wg)
# Simulation size along propagation direction
sim_length = 2 * straight_wg_length + 2 * bend_length + coup_length
# Spacing between waveguides and PML
sim_size = [
sim_length,
wg_spacing_in + wg_width + 2 * pml_spacing,
wg_height + 2 * pml_spacing,
]
# source
src_pos = sim_length / 2 - straight_wg_length / 2
# in-plane field monitor (optional, increases required data storage)
domain_monitor = td.FieldMonitor(
center=[0, 0, wg_height / 2], size=[td.inf, td.inf, 0], freqs=[freq0], name="field"
)
# initialize the simulation
sim = td.Simulation(
size=sim_size,
grid_spec=grid_spec,
structures=[oxide, coupler1, coupler2],
sources=[],
monitors=[domain_monitor],
run_time=50 / fwidth,
boundary_spec=td.BoundarySpec.all_sides(boundary=td.PML()),
)
[5]:
f, (ax1, ax2) = plt.subplots(1, 2, tight_layout=True, figsize=(15, 10))
ax1 = sim.plot(z=wg_height / 2, ax=ax1)
ax2 = sim.plot(x=src_pos, ax=ax2)
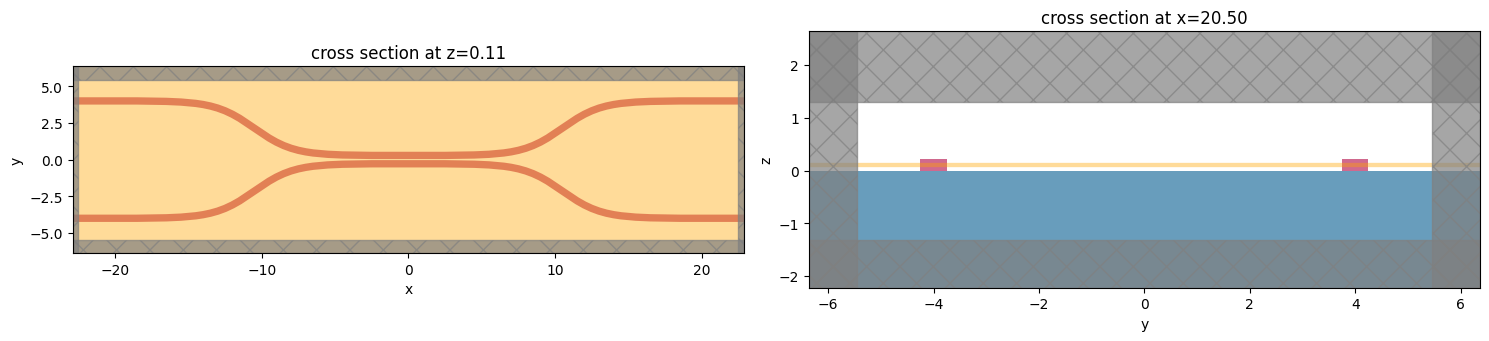
Setting up Scattering Matrix Tool#
Now, to use the S matrix tool, we need to defing the spatial extent of the βportsβ of our system using Port objects.
These ports will be converted into modal sources and monitors later, so they require both some mode specification and a definition of the direction that points into the system.
Weβll also give them names to refer to later.
[5]:
from tidy3d.plugins.smatrix.smatrix import Port
num_modes = 1
port_right_top = Port(
center=[src_pos, wg_spacing_in / 2, wg_height / 2],
size=[0, 4, 2],
mode_spec=td.ModeSpec(num_modes=num_modes),
direction="-",
name="right_top",
)
port_right_bot = Port(
center=[src_pos, -wg_spacing_in / 2, wg_height / 2],
size=[0, 4, 2],
mode_spec=td.ModeSpec(num_modes=num_modes),
direction="-",
name="right_bot",
)
port_left_top = Port(
center=[-src_pos, wg_spacing_in / 2, wg_height / 2],
size=[0, 4, 2],
mode_spec=td.ModeSpec(num_modes=num_modes),
direction="+",
name="left_top",
)
port_left_bot = Port(
center=[-src_pos, -wg_spacing_in / 2, wg_height / 2],
size=[0, 4, 2],
mode_spec=td.ModeSpec(num_modes=num_modes),
direction="+",
name="left_bot",
)
ports = [port_right_top, port_right_bot, port_left_top, port_left_bot]
Next, we will add the base simulation and ports to the ComponentModeler, along with the frequency of interest and a name for saving the batch of simulations that will get created later.
[6]:
from tidy3d.plugins.smatrix.smatrix import ComponentModeler
modeler = ComponentModeler(simulation=sim, ports=ports, freqs=[freq0], verbose=True, path_dir="data")
We can plot the simulation with all of the ports as sources to check things are set up correctly.
[8]:
f, (ax1, ax2) = plt.subplots(1, 2, tight_layout=True, figsize=(15, 10))
ax1 = modeler.plot_sim(z=wg_height / 2, ax=ax1)
ax2 = modeler.plot_sim(x=src_pos, ax=ax2)
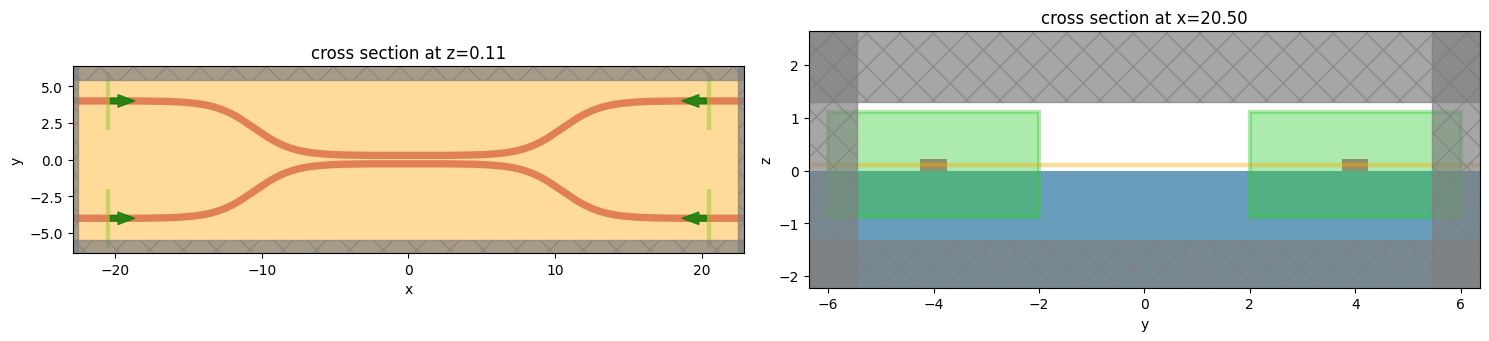
Solving for the S matrix#
With the component modeler defined, we may call itβs .solve()
method to run a batch of simulations to compute the S matrix. The tool will loop through each port and create one simulation per mode index (as defined by the mode specifications) where a unique modal source is injected. Each of the ports will also be converted to mode monitors to measure the mode amplitudes and normalization.
[7]:
smatrix = modeler.run()
14:51:32 PST Created task 'smatrix_right_top_0' with task_id 'fdve-d673cc21-f0ae-45b5-890d-53d561516b84' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbenc h?taskId=fdve-d673cc21-f0ae-45b5-890d-53d561516b84'.
14:51:33 PST Created task 'smatrix_right_bot_0' with task_id 'fdve-373f561c-3060-4cae-9008-6da23117e557' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbenc h?taskId=fdve-373f561c-3060-4cae-9008-6da23117e557'.
Created task 'smatrix_left_top_0' with task_id 'fdve-e4779888-4800-49e8-af12-51f712dbb184' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbenc h?taskId=fdve-e4779888-4800-49e8-af12-51f712dbb184'.
14:51:34 PST Created task 'smatrix_left_bot_0' with task_id 'fdve-611b08f2-8b4c-4bab-ad1b-4f50f703d3f4' and task_type 'FDTD'.
View task using web UI at 'https://tidy3d.simulation.cloud/workbenc h?taskId=fdve-611b08f2-8b4c-4bab-ad1b-4f50f703d3f4'.
14:51:37 PST Started working on Batch.
14:51:45 PST Maximum FlexCredit cost: 1.839 for the whole batch.
Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
14:51:46 PST Batch complete.
14:51:48 PST loading simulation from data/fdve-d673cc21-f0ae-45b5-890d-53d561516b84.hdf5
14:51:49 PST loading simulation from data/fdve-373f561c-3060-4cae-9008-6da23117e557.hdf5
14:51:51 PST loading simulation from data/fdve-e4779888-4800-49e8-af12-51f712dbb184.hdf5
14:51:52 PST loading simulation from data/fdve-611b08f2-8b4c-4bab-ad1b-4f50f703d3f4.hdf5
Working with Scattering Matrix#
The scattering matrix returned by the solve is an xr.DataArray relating the port names and mode_indices. For example smatrix.loc[dict(port_in=name1, mode_index_in=mode_index1, port_out=name2, mode_index_out=mode_index_2)]
gives the complex scattering matrix element.
For example:
[8]:
smatrix.loc[
dict(port_in="left_top", mode_index_in=0, port_out="right_bot", mode_index_out=0)
]
[8]:
<xarray.SMatrixDataArray (f: 1)> array([0.33469842-0.61911778j]) Coordinates: port_out <U9 'right_bot' port_in <U9 'left_top' mode_index_out int64 0 mode_index_in int64 0 * f (f) float64 1.934e+14
Alternatively, we can convert this into a numpy array:
[9]:
S = np.squeeze(smatrix.values)
print(S.shape)
(4, 4)
We can inspect S
and note that the diagonal elements are very small indicating low backscattering.
Summing each rows of the matrix should give 1.0 if no power was lost.
[12]:
np.sum(abs(S) ** 2, axis=0)
[12]:
array([0.98590153, 0.98590134, 0.98590724, 0.9859074 ])
There is a little power loss since the coupler was not optimized, most likely scattering from the bends and coupling region.
Finally, we can check whether S
is close to unitary as expected. S times itβs Hermitian conjugate should be the identy matrix.
[10]:
mat = S @ (np.conj(S.T))
[11]:
f, (ax1, ax2, ax3) = plt.subplots(1, 3, tight_layout=True, figsize=(10, 3.5))
imabs = ax1.matshow(abs(mat))
vmax = np.abs(mat.real).max()
imreal = ax2.matshow(mat.real, cmap="RdBu", vmin=-vmax, vmax=vmax)
vmax = np.abs(mat.imag).max()
imimag = ax3.matshow(mat.imag, cmap="RdBu", vmin=-vmax, vmax=vmax)
ax1.set_title("$|S^\dagger S|$")
ax2.set_title("$\Re\{S^\dagger S\}$")
ax3.set_title("$\Im\{S^\dagger S\}$")
plt.colorbar(imabs, ax=ax1)
plt.colorbar(imreal, ax=ax2)
plt.colorbar(imimag, ax=ax3)
ax1.grid(False)
ax2.grid(False)
ax3.grid(False)
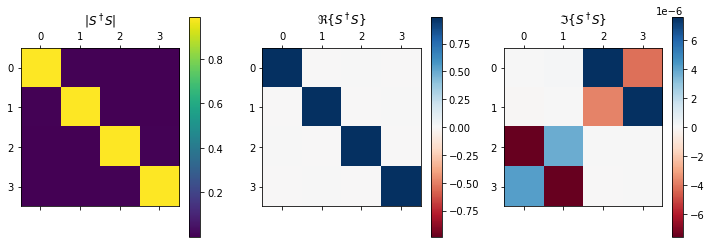
It looks pretty close, but there seems to indeed be a bit of loss (expected).
Viewing individual Simulation Data#
To verify, we may want to take a look the individual simulation data. For that, we can load up the batch and inspect the SimulationData for each task.
[15]:
f, (ax1, ax2) = plt.subplots(2, 1, tight_layout=True, figsize=(15, 10))
ax1 = modeler.batch.load(path_dir="data")["smatrix_left_top_0"].plot_field(
"field", field_name="E", val="abs^2", z=wg_height / 2, ax=ax1
)
ax2 = modeler.batch.load(path_dir="data")["smatrix_right_bot_0"].plot_field(
"field", field_name="E", val="abs^2", z=wg_height / 2, ax=ax2
)
WARNING: 'int' field name is deprecated and will be sim_data.py:497 removed in the future. Plese use field_name='E' and val='abs^2' for the same effect.
WARNING: 'int' field name is deprecated and will be sim_data.py:497 removed in the future. Plese use field_name='E' and val='abs^2' for the same effect.
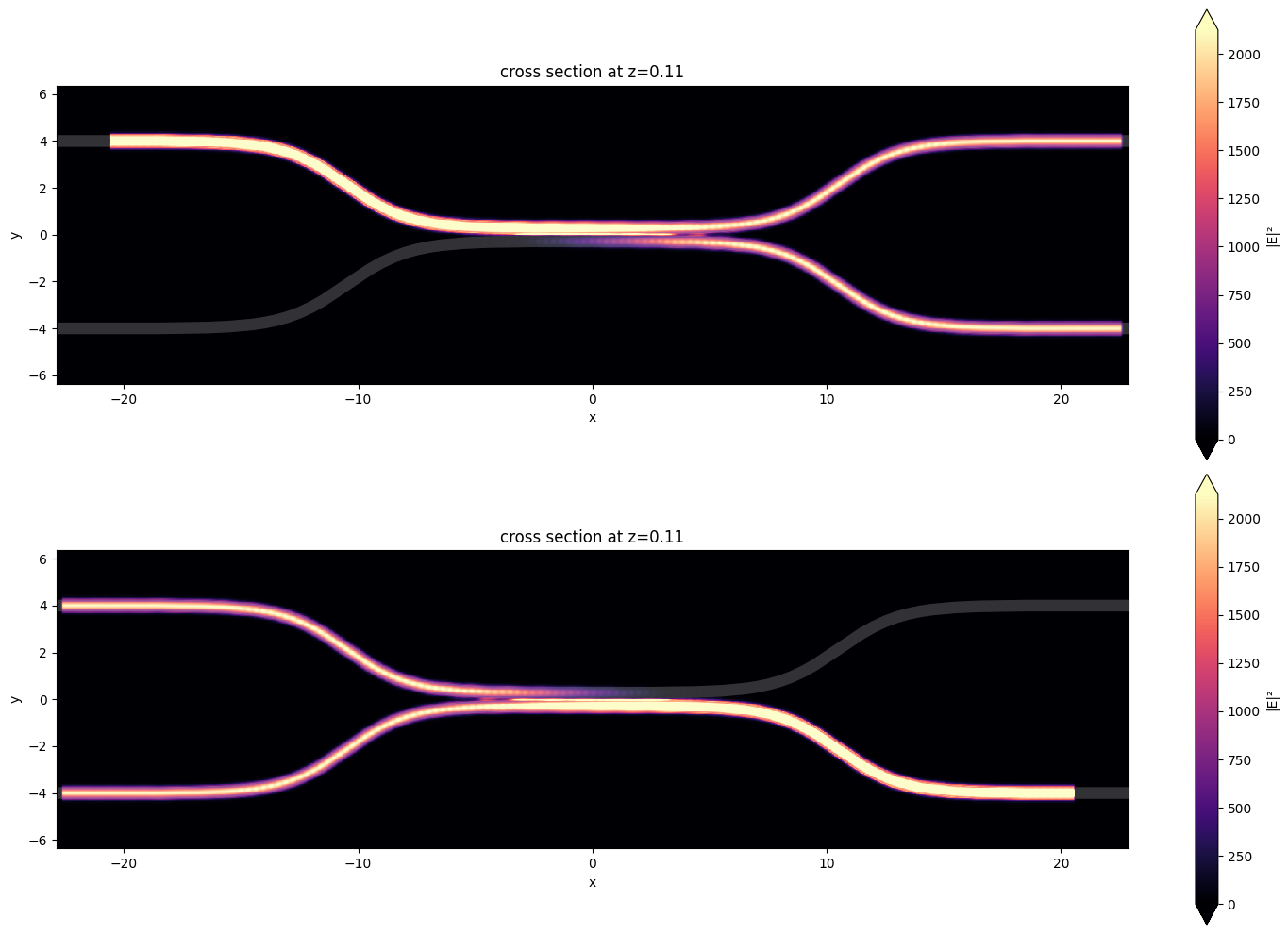
Saving and Loading Results#
Finally, we can save and load the component modeler from file to save the results.
[16]:
fname = "data/modeler.json"
modeler.to_file(fname)
modeler2 = ComponentModeler.from_file(fname)
f, (ax1, ax2) = plt.subplots(2, 1, tight_layout=True, figsize=(15, 10))
ax1 = modeler2.batch.load(path_dir="data")["smatrix_left_top_0"].plot_field(
"field", "int", z=wg_height / 2, ax=ax1
)
ax2 = modeler2.batch.load(path_dir="data")["smatrix_right_bot_0"].plot_field(
"field", "int", z=wg_height / 2, ax=ax2
)
WARNING: 'int' field name is deprecated and will be sim_data.py:497 removed in the future. Plese use field_name='E' and val='abs^2' for the same effect.
WARNING: 'int' field name is deprecated and will be sim_data.py:497 removed in the future. Plese use field_name='E' and val='abs^2' for the same effect.
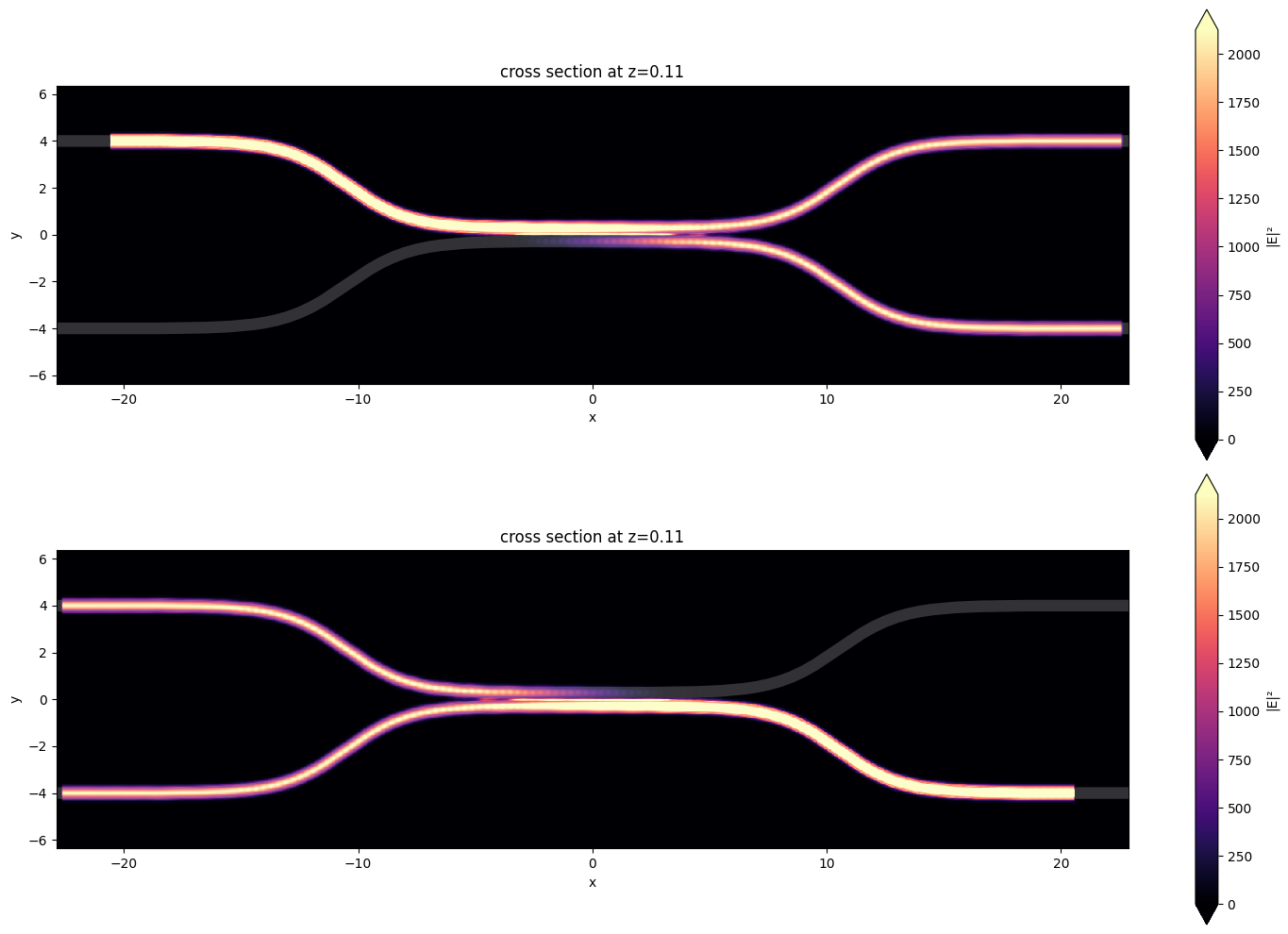
Element Mappings#
If we wish, we can specify mappings between scattering matrix elements that we want to be equal up to a multiplicative factor. We can define these as element_mappings
in the ComponentModeler
.
βIndicesβ are defined as a tuple of (port_name: str, mode_index: int)
βElementsβ are defined as a tuple of output and input indices, respectively.
The element mappings are therefore defined as a tuple of (element, element, value)
where the second element
is set by the value of the 1st element
times the supplied value
.
As an example, letβs define this element mapping from the example above to enforce that the coupling between bottom left to bottom right should be equal to the coupling between top left to top right.
[17]:
# these are the "indices" in our scattering matrix
left_top = ("left_top", 0)
right_top = ("right_top", 0)
left_bot = ("left_bot", 0)
right_bot = ("right_bot", 0)
# we define the scattering matrix elements coupling the top ports and bottom ports as pairs of these indices
top_coupling_r2l = (left_top, right_top)
bot_coupling_r2l = (left_bot, right_bot)
top_coupling_l2r = (right_top, left_top)
bot_coupling_l2r = (right_bot, left_bot)
# map the top coupling to the bottom coupling with a multiplicative factor of +1
map_horizontal_l2r = (top_coupling_l2r, bot_coupling_l2r, +1)
map_horizontal_r2l = (top_coupling_r2l, bot_coupling_r2l, +1)
element_mappings = (map_horizontal_l2r, map_horizontal_r2l)
[18]:
# run the component modeler again
modeler = ComponentModeler(
simulation=sim,
ports=ports,
freqs=[freq0],
element_mappings=element_mappings,
verbose=True,
path_dir="data"
)
smatrix = modeler.run()
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- 4ae19a0b-6656-4767-b012-1dc3e17e3dfdv1'.
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- 6609948e-9edb-4cfd-9132-8c0de1e12b27v1'.
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- 458d2239-0779-4d48-92b1-f7c0d021609ev1'.
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- ccc9701f-20f7-4937-a40b-bff4b9e7e42bv1'.
[16:24:48] Started working on Batch. container.py:475
[16:25:12] Maximum FlexCredit cost: 1.763 for the whole batch. container.py:479 Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
[16:28:11] Batch complete. container.py:522
The resulting scattering matrix will have the element mappings applied, we can check this explicitly.
[19]:
# assert that the horizontal couping elements are exactly equal
LT_RT = np.squeeze(smatrix.loc[dict(port_in="left_top", port_out="right_top")])
LB_RB = np.squeeze(smatrix.loc[dict(port_in="left_bot", port_out="right_bot")])
print(f"top to top coupling = {LT_RT:.5f}")
print(f"bottom to bottom coupling = {LB_RB:.5f}")
assert np.isclose(LT_RT, LB_RB)
top to top coupling = -0.61725-0.32981j
bottom to bottom coupling = -0.61725-0.32981j
Incomplete Scattering Matrix#
Finally, to exclude some rows of the scattering matrix, one can supply a run_only
paramteter to the ComponentModeler
.
run_only
contains the scattering matrix indices that the user wants to run as a source. If any indices are excluded, they will not be run.
For example, if one wants to compute scattering matrix elements from only the ports on the left hand side, the run_only
could be defined as follows.
[20]:
run_only = (left_top, left_bot)
modeler = ComponentModeler(
simulation=sim, ports=ports, freqs=[freq0], run_only=run_only, verbose=True, path_dir="data"
)
smatrix = modeler.run()
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- f39c4ead-9fac-421b-abbf-60900dcbde93v1'.
View task using web UI at webapi.py:190 'https://tidy3d.simulation.cloud/workbench?taskId=fdve- 5dfff954-b1b1-4089-92a3-bb2e14376305v1'.
[16:28:42] Started working on Batch. container.py:475
[16:28:51] Maximum FlexCredit cost: 0.881 for the whole batch. container.py:479 Use 'Batch.real_cost()' to get the billed FlexCredit cost after the Batch has completed.
[16:30:16] Batch complete. container.py:522
The resulting scattering matrix will have all zeros for elements corresponding to ports not included in the in run_only
inputs.
[21]:
s_matrix_left_top = np.squeeze(smatrix.loc[dict(port_in="left_top")].values)
print("output from run_only port : \n", s_matrix_left_top)
assert "right_top" not in smatrix.coords["port_in"]
output from run_only port :
[-0.61725057-0.32980806j 0.33469665-0.61911863j 0.00119965+0.02065445j
-0.00907913-0.01718773j]
[ ]: